How To Build a Simple Featured Calculator using Python
In this tutorial, we will create a basic calculator using Tkinter, the standard GUI toolkit for Python. This calculator will perform basic arithmetic operations such as addition, subtraction, multiplication, and division, as well as some additional functions like square root, square, percentage, and backspace.
Let's go through the code and understand how each component works.
import tkinter as tk
from tkinter import ttk
import math
Here, we import the necessary modules. Tkinter is used for creating the GUI, ttk for themed widgets, and math for mathematical operations.
def on_click(button_value):
current_text = result_var.get()
if button_value == 'C':
result_var.set('')
elif button_value == '=':
try:
result_var.set(eval(current_text))
except Exception as e:
result_var.set('Error')
elif button_value == 'sqrt':
try:
result_var.set(math.sqrt(float(current_text)))
except Exception as e:
result_var.set('Error')
elif button_value == 'x^2':
try:
result_var.set(eval(current_text) ** 2)
except Exception as e:
result_var.set('Error')
elif button_value == '%':
try:
result_var.set(eval(current_text) / 100)
except Exception as e:
result_var.set('Error')
elif button_value == '⌫':
result_var.set(current_text[:-1])
else:
result_var.set(current_text + str(button_value))
The on_click
function is called whenever a button is pressed. It handles different button values and performs corresponding actions like clearing the display, evaluating the expression, calculating square root and square, handling percentages, and handling backspace.
# Create the main window
window = tk.Tk()
window.title("Subham's Calculator")
We create the main window for the calculator and set its title.
# Variable to hold the current input/result
result_var = tk.StringVar()
# Variable to hold the current operation being performed
operation_var = tk.StringVar()
Two StringVar
variables are created to hold the current input/result and the current operation being performed.
# Style configuration
style = ttk.Style()
style.configure("TButton", padding=10, font=('Arial', 12))
We configure the style for the buttons to make them visually appealing.
# Entry widget to display the current input/result
entry = ttk.Entry(window, textvariable=result_var, justify='right', font=('Arial', 14))
entry.grid(row=0, column=0, columnspan=4, sticky="nsew")
# Label to display the current operation
operation_label = ttk.Label(window, textvariable=operation_var, font=('Arial', 10))
operation_label.grid(row=1, column=0, columnspan=4, sticky="nsew")
An entry widget is created to display the current input/result, and a label is created to display the current operation.
# Define the buttons
buttons = [
'7', '8', '9', '/',
'4', '5', '6', '*',
'1', '2', '3', '-',
'0', '.', 'C', '+',
'sqrt', 'x^2', '%', '⌫',
'(', ')', '='
]
A list of button values is defined for creating the calculator buttons.
# Create and place the buttons in the grid
row_val = 2
col_val = 0
for button in buttons:
ttk.Button(window, text=button, style="TButton",
command=lambda b=button: on_click(b)).grid(row=row_val, column=col_val, sticky="nsew")
col_val += 1
if col_val > 3:
col_val = 0
row_val += 1
Buttons are created using the values from the list, and each button is associated with the on_click
function.
# Configure row and column weights
for i in range(2, 7):
window.grid_columnconfigure(i, weight=1)
window.grid_rowconfigure(i, weight=1)
Row and column weights are configured to allow resizing of the window.
# Start the GUI event loop
window.mainloop()
Finally, the GUI event loop is started, allowing the user to interact with the calculator.
Feel free to run this code and explore the functionality of the calculator!
FullCode
import tkinter as tk
from tkinter import ttk
import math
def on_click(button_value):
current_text = result_var.get()
if button_value == 'C':
result_var.set('')
elif button_value == '=':
try:
result_var.set(eval(current_text))
except Exception as e:
result_var.set('Error')
elif button_value == 'sqrt':
try:
result_var.set(math.sqrt(float(current_text)))
except Exception as e:
result_var.set('Error')
elif button_value == 'x^2':
try:
result_var.set(eval(current_text) ** 2)
except Exception as e:
result_var.set('Error')
elif button_value == '%':
try:
result_var.set(eval(current_text) / 100)
except Exception as e:
result_var.set('Error')
elif button_value == '⌫':
result_var.set(current_text[:-1])
else:
result_var.set(current_text + str(button_value))
# Create the main window
window = tk.Tk()
window.title("Subham's Calculator")
# Variable to hold the current input/result
result_var = tk.StringVar()
# Variable to hold the current operation being performed
operation_var = tk.StringVar()
# Style configuration
style = ttk.Style()
style.configure("TButton", padding=10, font=('Arial', 12))
# Entry widget to display the current input/result
entry = ttk.Entry(window, textvariable=result_var, justify='right', font=('Arial', 14))
entry.grid(row=0, column=0, columnspan=4, sticky="nsew")
# Label to display the current operation
operation_label = ttk.Label(window, textvariable=operation_var, font=('Arial', 10))
operation_label.grid(row=1, column=0, columnspan=4, sticky="nsew")
# Define the buttons
buttons = [
'7', '8', '9', '/',
'4', '5', '6', '*',
'1', '2', '3', '-',
'0', '.', 'C', '+',
'sqrt', 'x^2', '%', '⌫',
'(', ')', '='
]
# Create and place the buttons in the grid
row_val = 2
col_val = 0
for button in buttons:
ttk.Button(window, text=button, style="TButton",
command=lambda b=button: on_click(b)).grid(row=row_val, column=col_val, sticky="nsew")
col_val += 1
if col_val > 3:
col_val = 0
row_val += 1
# Configure row and column weights
for i in range(2, 7):
window.grid_columnconfigure(i, weight=1)
window.grid_rowconfigure(i, weight=1)
# Start the GUI event loop
window.mainloop()
Output
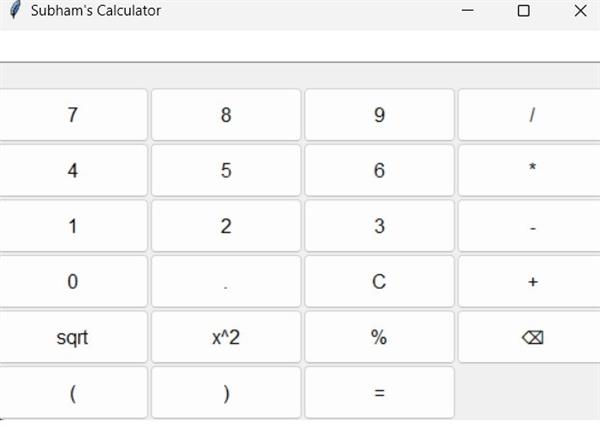