Blazor route parameters
Blazor Route Parameters
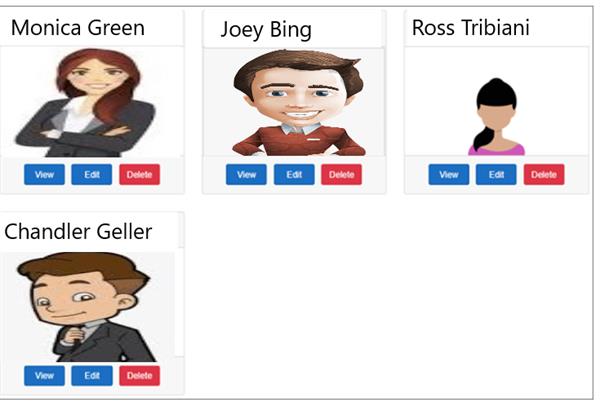
At the point when we click the View button, the client ought to be diverted to the URL (/employeedetails/3). The worth 3 in the URI is the employee id. The blazor segment (EmployeeDetails) shows the subtleties of the Employee.
EmployeeDetails.razor
To get to this segment, employee Id must be passed in the URL as a course boundary. This is determined by utilizing the @page order course format.
@page "/employeedetails/{Id}"
EmployeeDetailsBase.cs
The Id open property is designed with the [Parameter] quality.
The name of the property (Id) matches with the name of the course boundary in the @page order (@page "/employeedetail/{Id}")
The blazor switch utilizes the course boundary esteem in the URL to populate the Id property in the segment class.
The accompanying 2 conditions must be valid, for the switch to have the option to plan the course boundary esteem in the URL to the open property in the part class
The open property in the segment class must be enlivened with [Parameter] characteristics.
The name of the property in the segment class and the name of the course boundary in the @page order must be the equivalent.
In the event that the names are distinctive, you will get the accompanying runtime special case
InvalidOperationException: Object of type 'EmployeeManagement.Web.Pages.EmployeeDetail' doesn't have a property coordinating the name 'Id'.
Blazor discretionary Route Parameter
Discretionary boundaries aren't upheld. Nonetheless, we can utilize two @page orders to get the impact of discretionary boundaries.
@page "/employeedetails"
@page "/employeedetails/{Id}"
The principal @page order permits route to the segment without a boundary. The second @page order gets the {Id} course boundary and relegates the incentive to the Id open property on the part class.
public class EmployeeDetailsBase : ComponentBase
{
public Employee Employee { get; set; } = new Employee();
[Inject]
public IEmployeeService EmployeeService { get; set; }
[Parameter]
public string Id { get; set; }
protected override async Task OnInitializedAsync()
{
// If Id value is not supplied in the URL, use the value 1
Id = Id ?? "1";
Employee = await EmployeeService.GetEmployee(int.Parse(Id));
}
}
Building URL with route parameter
On the rundown page when the View button is clicked we need to divert the client to/employeedetail/id.
IEmployeeService.cs
EmployeeService.cs
public class EmployeeService : IEmployeeService
{
private readonly HttpClient httpClient;
public EmployeeService(HttpClient httpClient)
{
this.httpClient = httpClient;
}
public async Task<Employee> GetEmployee(int id)
{
return await httpClient.GetJsonAsync<Employee>($"api/employees/{id}");
}
public async Task<IEnumerable<Employee>> GetEmployees()
{
return await httpClient.GetJsonAsync<Employee[]>("api/employees");
}
}