What is VueJs Rendering | VueJs Tutorials
What is VueJs Rendering | VueJs Tutorials
Vue recommends utilizing templates to build your HTML in by far most of the cases. There are circumstances, however, where you truly need the full programmatic power of JavaScript. That's where you can utilize the render function, a closer-to-the-compiler alternative to templates.
In conditional rendering, we will discuss using if, if-else, if-else-if, show, etc. In list rendering, we will discuss how to use for loop.
Conditional Rendering
Let's begin and work on a model first to clarify the details for conditional rendering. With conditional rendering, we need to output only when the condition is met and the conditional check is done with the assistance of if, if-else, if-else-if, show, and so on.
v-if
Example
<html>
<head>
<title>VueJs Instance</title>
<script type = "text/javascript" src = "js/vue.js"></script>
</head>
<body>
<div id = "databinding">
<button v-on:click = "showdata" v-bind:style = "styleobj">Click Me</button>
<span style = "font-size:25px;"><b>{{show}}</b></span>
<h1 v-if = "show">h1 tag</h1>
<h2>h2 tag</h2>
</div>
<script type = "text/javascript">
var vm = new Vue({
el: '#databinding',
data: {
show: true,
styleobj: {
backgroundColor: '#2196F3!important',
cursor: 'pointer',
padding: '8px 16px',
verticalAlign: 'middle',
}
},
methods : {
showdata : function() {
this.show = !this.show;
}
},
});
</script>
</body>
</html>
Output
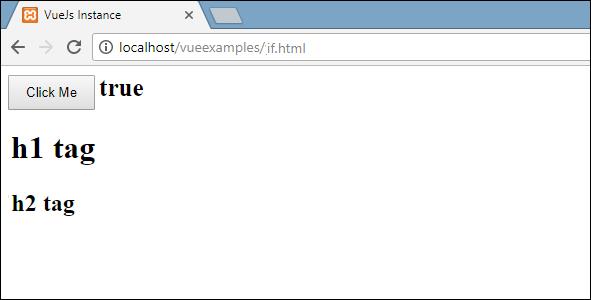
In the above example, we have created a button and two h1 tags with the message.
A variable called show is declared and initialized to value true. It is shown near the button. On the click of the button, we are calling a method show data, which toggles the value of the variable show. This implies on the click of the button, the value of the variable show will change from true to false and false to true.
We have assigned it to the h1 tag as shown in the following code snippet.
<button v-on:click = "showdata" v-bind:style = "styleobj">Click Me</button>
<h1 v-if = "show">h1 tag</h1>
So, what it will do is, it will check the value of the variable show, and if it's true the h1 tag will be displayed. Click the button and view in the browser, as the value of the show variable changes to false, the h1 tag isn't shown in the browser. It is displayed only when the show variable is true.
The following is the display in the browser.
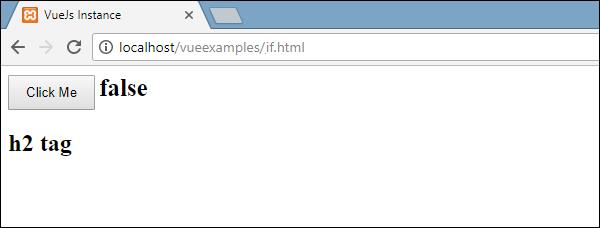
If we check in the browser, this is what we get when the show is false.
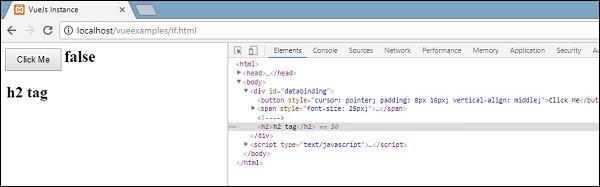
The h1 tag is removed from the DOM when the variable show is set to false.
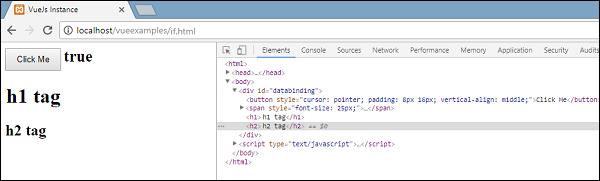
This is what we see when the variable is true. The h1 tag is added back to the DOM when the variable show is set to true.
v-else
In the following example, we have added v-else to the second h1 tag.
Example
<html>
<head>
<title>VueJs Instance</title>
<script type = "text/javascript" src = "js/vue.js"></script>
</head>
<body>
<div id = "databinding">
<button v-on:click = "showdata" v-bind:style = "styleobj">Click Me</button>
<span style = "font-size:25px;"><b>{{show}}</b></span>
<h1 v-if = "show">h1 tag</h1>
<h2 v-else>h2 tag</h2>
</div>
<script type = "text/javascript">
var vm = new Vue({
el: '#databinding',
data: {
show: true,
styleobj: {
backgroundColor: '#2196F3!important',
cursor: 'pointer',
padding: '8px 16px',
verticalAlign: 'middle',
}
},
methods : {
showdata : function() {
this.show = !this.show;
}
},
});
</script>
</body>
</html>
v-else is added using the following code snippet.
<h1 v-if = "show">h1 tag</h1>
<h2 v-else>h2 tag</h2>
Now, if the show is true “This is h1 tag” will be displayed, and if false “h2 tag” will be displayed. This is what we will get in the browser.
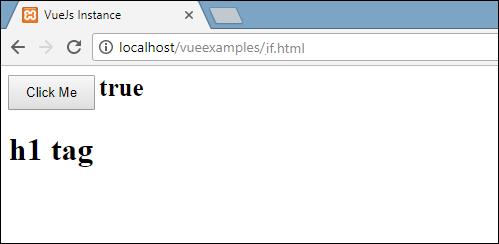
The above presentation is the point at which the show variable is valid. Since we have included v-else, the second statement is absent. Now, when we click the button the show variable will turn out to be false and the second statement will be displayed as shown in the accompanying screen capture.
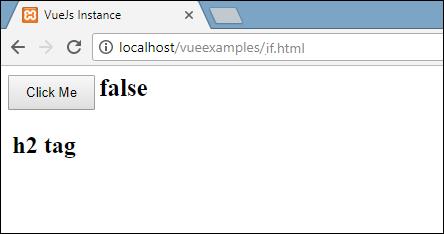
v-show
v-show acts the same as v-if. It also shows and hides the elements dependent on the condition assigned to it. The difference between v-if and v-show is that v-if eliminates the HTML element from the DOM if the condition is false, and includes it back if the condition is true. Though v-show hides the element, if the condition is false with display: none. It shows the element back if the condition is true. Hence, the element is available in the dom always.
Example
<html>
<head>
<title>VueJs Instance</title>
<script type = "text/javascript" src = "js/vue.js"></script>
</head>
<body>
<div id = "databinding">
<button v-on:click = "showdata" v-bind:style = "styleobj">Click Me</button>
<span style = "font-size:25px;"><b>{{show}}</b></span>
<h1 v-if = "show">h1 tag</h1>
<h2 v-else>h2 tag</h2>
<div v-show = "show">
<b>V-Show:</b>
<img src = "images/ichigo.jpg" width = "100" height = "100" />
</div>
</div>
<script type = "text/javascript">
var vm = new Vue({
el: '#databinding',
data: {
show: true,
styleobj: {
backgroundColor: '#2196F3!important',
cursor: 'pointer',
padding: '8px 16px',
verticalAlign: 'middle',
}
},
methods : {
showdata : function() {
this.show = !this.show;
}
},
});
</script>
</body>
</html>
v-show is assigned to the HTML element using the following code snippet.
<div v-show = "show"><b>V-Show:</b><img src = "images/img.jpg" width = "100" height = "100" /></div>
We have used the same variable show and based on it being true/false, the image is displayed in the browser.
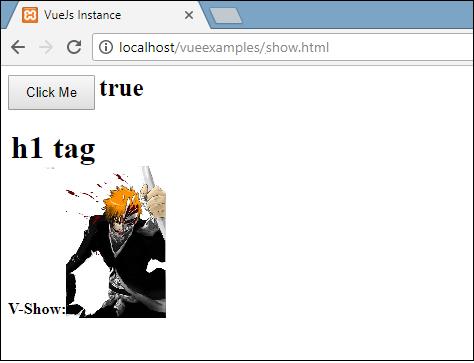
Now, since the variable show is true, the image is as displayed in the above screenshot. Let us click the button and see the display.
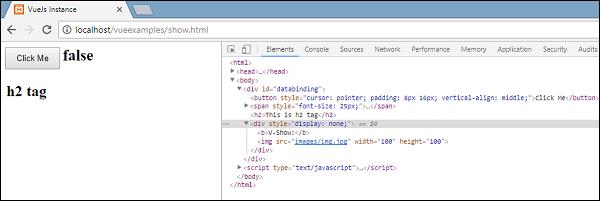
The variable show is false, hence the image is hidden. If we inspect and see the element, the div along with the image is still a part of the DOM with the style property display: none as seen in the above screenshot.
List Rendering
v-for
Let us now discuss list rendering with the v-for directive.
Example
<html>
<head>
<title>VueJs Instance</title>
<script type = "text/javascript" src = "js/vue.js"></script>
</head>
<body>
<div id = "databinding">
<input type = "text" v-on:keyup.enter = "showinputvalue"
v-bind:style = "styleobj" placeholder = "Enter Fruits Name"/>
<h1 v-if = "items.length>0">Display Fruits Name</h1>
<ul>
<li v-for = "a in items">{{a}}</li>
</ul>
</div>
<script type = "text/javascript">
var vm = new Vue({
el: '#databinding',
data: {
items:[],
styleobj: {
width: "30%",
padding: "12px 20px",
margin: "8px 0",
boxSizing: "border-box"
}
},
methods : {
showinputvalue : function(event) {
this.items.push(event.target.value);
}
},
});
</script>
</body>
</html>
A variable called things is declared as an array. In methods, there is a method called showinputvalue, which is assigned to the input box that takes the names of the fruits. In the method, the fruits entered inside the textbox are added to the array utilizing the accompanying piece of code.
showinputvalue : function(event) {
this.items.push(event.target.value);
}
We have used v-for to display the fruits entered as in the following piece of code. V-for helps to iterate over the values present in the array.
<ul>
<li v-for = "a in items">{{a}}</li>
</ul>
To iterate over the array with for loop, we have to use v-for = ”a in items” where a holds the values in the array and will display till all the items are done.
Output
The following is the output in the browser.
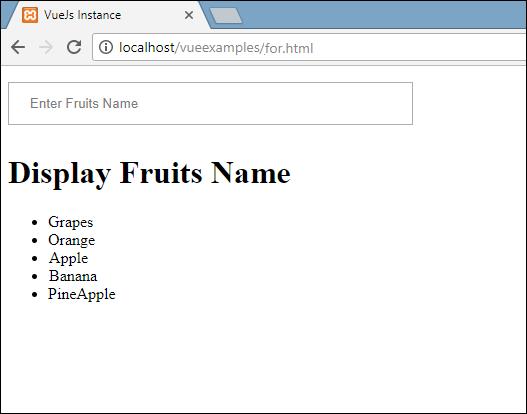
On inspecting the items, this is what it shows in the browser. In the DOM, we don’t see any v-for directive to the li element. It displays the DOM without any VueJS directives.
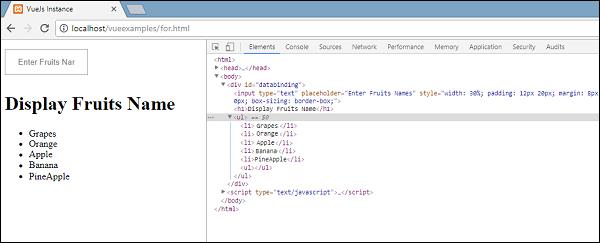
If we wish to display the index of the array, it is done using the following code.
<html>
<head>
<title>VueJs Instance</title>
<script type = "text/javascript" src = "js/vue.js"></script>
</head>
<body>
<div id = "databinding">
<input type = "text" v-on:keyup.enter = "showinputvalue"
v-bind:style = "styleobj" placeholder = "Enter Fruits Names"/>
<h1 v-if = "items.length>0">Display Fruits Name</h1>
<ul>
<li v-for = "(a, index) in items">{{index}}--{{a}}</li>
</ul>
</div>
<script type = "text/javascript">
var vm = new Vue({
el: '#databinding',
data: {
items:[],
styleobj: {
width: "30%",
padding: "12px 20px",
margin: "8px 0",
boxSizing: "border-box"
}
},
methods : {
showinputvalue : function(event) {
this.items.push(event.target.value);
}
},
});
</script>
</body>
</html>
To get the index, we have added one more variable in the bracket as shown in the following piece of code.
<li v-for = "(a, index) in items">{{index}}--{{a}}</li>
In (a index), a is the value and index is the key. The browser display will now be as shown in the following screenshot. Thus, with the help of index, any specific values can be displayed.
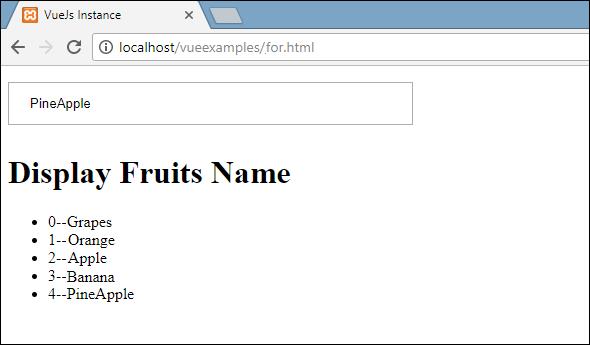