Defining Variables
Structure definitions do not reserve any space in memory; rather, each definition creates a new data type that is used to define variables. Structure variables are defined as variables of other types.
The definition
struct card aCard, deck[ 52 ], *cardPtr;
declares aCard to be a variable of type struct card, declares deck to be an array with 52 elements of type struct card and declares cardPtr to be a pointer to struct card. Variables of a given structure type may also be declared by placing a comma-separated list of the variable names between the closing brace of the structure definition and the semicolon that ends the structure definition.
For example, the preceding definition could have been incorporated into the struct card structure defined as follows:
struct card {
char *face;
char *suit;
} aCard, deck[ 52 ], *cardPtr;
Structure Tag Names
The structure tag name is optional. If a structure definition does not contain a structure tag name, variables of the structure type may be declared only in the structure definition, not in a separate declaration.
Choosing a meaningful structure tag name helps make a program self-documenting.
Operations That Can Be Performed on Structures
The only valid operations that may be performed on structures are the following: assigning structure variables to structure variables of the same type, taking the address (&) of a structure variable, accessing the members of a structure variable and using the sizeof operator to determine the size of a structure variable.
Assigning a structure of one type to a structure of a different type is a compilation error. Structures may not be compared using operators == and !=, because structure members are not necessarily stored in consecutive bytes of memory. Sometimes there are “holes” in a structure, because computers may store specific data types only on certain memory boundaries such as half word, word or double word boundaries.
A word is a standard memory unit used to store data in a computer usually 2 bytes or 4 bytes. Consider the following structure definition, in which sample1 and sample2 of type struct example are declared:
struct example {
char c;
int i;
} sample1, sample2;
A computer with 2-byte words may require that each member of struct example be aligned on a word boundary, i.e., at the beginning of a word (this is machine dependent).
The figure shows a sample storage alignment for a variable of type struct example that has been assigned the character 'a' and the integer 97 (the bit representations of the values are shown). If the members are stored beginning at word boundaries, there is a 1-byte hole (byte 1 in the figure) in the storage for variables of type struct example.
The value of the 1-byte hole is undefined. Even if the member values of sample1 and sample2 are in fact equal, the structures are not necessarily equal, because the undefined 1-byte holes are not likely to contain identical values.
Figure
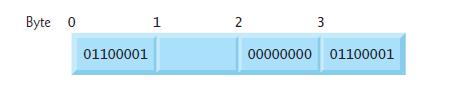