What is React Constructor? | React Constructor | React Tutorial
What is React Constructor? | React Constructor | React Tutorial
React Constructor:
The constructor is a way to initialize an object's state in a class. Constructor automatically called during the creation of an object in a class.
The concept of a constructor is the same as we know. Here, The constructor in a React component is called before the component is mounted. We need to call the super(props) method before any other statement during the implementation of the constructor for a React component. this.props will be undefined in the constructor and can lead to bugs, If we don't call the super(props) method.
Syntax:
Constructor(props){
super(props);
}
In React, we mainly use constructors for two purposes:
- Constructor used for initializing the local state of the component by assigning an object to this.state.
- Constructor used for binding event handler methods that occur in your component.
We cannot call setState() method directly in the constructor(). If the component needs to use a local state, we need directly to use 'this.state' to assign the initial state in the constructor. It only uses this.state to assign the initial state. All other methods need to use set.state() method.
Example
We can understand the concept of constructor from the below example.
App.js
import React, { Component } from 'react';
class App extends Component {
constructor(props){
super(props);
this.state = {
data: 'www.tutorialslink.com'
}
this.handleEvent = this.handleEvent.bind(this);
}
handleEvent(){
console.log(this.props);
}
render() {
return (
<div className="App">
<h2>React Constructor Example</h2>
<input type ="text" value={this.state.data} />
<button onClick={this.handleEvent}>Please Click</button>
</div>
);
}
}
export default App;
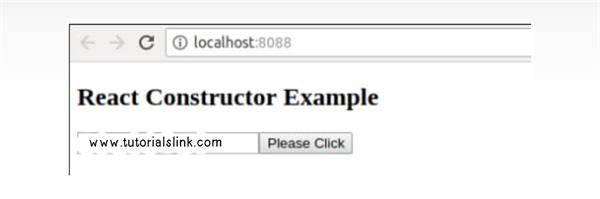
Some common question related to the constructor are:
1. To have a constructor in every component is necessary?
It's not necessary to have a constructor in every component. It simply returns a node, If the component is not complex.
class App extends Component {
render () {
return (
<p> Name: { this.props.name }</p>
);
}
}
2. To call super() inside a constructor is necessary?
Yes, To call super() inside a constructor is necessary. you need to call super(), If you need to set a property or access 'this' inside the constructor in your component.
class App extends Component {
constructor(props){
this.fName = "Jhon"; // 'this' is not allowed before super()
}
render () {
return (
<p> Name: { this.props.name }</p>
);
}
}
After running the above code, we will get an error saying 'this' is not allowed before super(). So if we need to access the props inside the constructor, we need to call super(props).
Arrow Functions
This is the new feature of the ES6 standard. If you want to use arrow functions, then it's not necessary to bind any event to 'this.' The scope of 'this' is global and not limited to any calling function. There is no need to bind 'this' inside the constructor, so If you are using Arrow Function.
import React, { Component } from 'react';
class App extends Component {
constructor(props){
super(props);
this.state = {
data: 'www.tutorilaslink.com'
}
}
handleEvent = () => {
console.log(this.props);
}
render() {
return (
<div className="App">
<h2>React Constructor Example</h2>
<input type ="text" value={this.state.data} />
<button onClick={this.handleEvent}>Please Click</button>
</div>
);
}
}
export default App;
In the following ways we can use a constructor :
1) To initialize the state constructor is used.
class App extends Component {
constructor(props){
// here, it is setting initial value for 'inputTextValue'
this.state = {
inputTextValue: 'initial value',
};
}
}
2) Using 'this' keyword inside the constructor
class App extends Component {
constructor(props) {
// when you use 'this' in constructor, super() needs to be called first
super();
// it means, when you want to use 'this.props' in constructor, call it as below
super(props);
}
}
3) Initializing third-party libraries
class App extends Component {
constructor(props) {
this.myBook = new MyBookLibrary();
//Here, you can access props without using 'this'
this.Book2 = new MyBookLibrary(props.environment);
}
}
4) Binding some context(this) when we need a class method to be passed in props to children.
class App extends Component {
constructor(props) {
// when you need to 'bind' context to a function
this.handleFunction = this.handleFunction.bind(this);
}
}