What do you mean by React Component API? | React Component API | React Tutorial
React Component API | React Tutorial
React Component API doesn’t replace class components. It simply offers a different method for creating components. And It has drawn the attention of many developers away from the classic class components.
React Component API provides functions for:
- state changes
- lifecycle stages
- rendering
The below methods are the methods you can call from your components.
Here we have discussed just two of them: setState() and forceUpdate().
1. setState()
In React, the setState() method enqueues changes to the component state and tells React that this component and the children need to be re-rendered with the updated state. This is the primary function you use to update the user interface in response to event handlers and server responses. But the component does not always immediately update.
Syntax
this.stateState(object newState[, function callback]);
In the above syntax, there is an optional callback function that is executed once setState() is completed and the component is re-rendered. If we want, We can optionally pass an object as the first argument to setState() instead of a function.
Example
App.js
import React, { Component } from 'react';
import PropTypes from 'prop-types';
class App extends React.Component {
constructor() {
super();
this.state = {
msg: "Hello Developer!"
};
this.updateSetState = this.updateSetState.bind(this);
}
updateSetState() {
this.setState({
msg:"Welcome in Tutorials Link.This Website has awesome contents for CS tutorials."
});
}
render() {
return (
<div>
<h1>{this.state.msg}</h1>
<button onClick = {this.updateSetState}>Check Set State</button>
<h1>{this.name}</h1>
</div>
);
}
}
export default App;
main.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App.js';
ReactDOM.render(<App/>, document.getElementById('app'));
output:
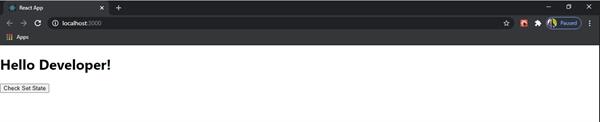

2. forceUpdate()
Usually, whenever your component’s state or props change, your component will re-render. React has a forceUpdate() function by using that we can force the react component to re-render. If the render() method depends on some other data, then you can tell React that the component needs re-rendering by calling forceUpdate(). Usually, you should try to avoid all uses of forceUpdate() and only read from this.props and this.state in render().
Syntax
Syntax
Component.forceUpdate(callback);
Example
App.js
import React, { Component } from 'react';
import PropTypes from 'prop-types';
class App extends React.Component {
handleUpdate = () => {
this.forceUpdate();
};
render() {
return (
<div>
<h1>Random Number Generator</h1>
<h1>{Math.random()}</h1>
<button onClick={this.handleUpdate}>Update</button>
</div>
);
}
}
export default App;
main.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App.js';
ReactDOM.render(<App/>, document.getElementById('app'));
Output:
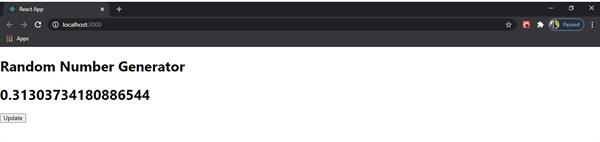
We can see, whenever you click on the Update button, it'll generate a random number automatically. It can be shown in the below image the usage of forceUpdate().