Music App with JavaScript | JavaScript
Music App with JavaScript | JavaScript
With the help of different types of events, functions, methods, classes, styling, and more with Vanilla JavaScript, HTML, and CSS we can create a music app of our own. A little bit of knowledge on event and event handling from JavaScript can make your work easier.
There is a need to set up 3 things:
- The HTML file
- The CSS file
- The JavaScript file
but as it is a music app and it will require some additional folders such as:
- Images
- Music
The above folders will have album images and music files of songs respectively.
One last thing we have to add is a small library of icons that will be needed for app buttons. Just click the link here, the zip folder will download and unzip it, extract the icon folder then simply drag and drop in the project.
HTML Part:
The skeleton of the Music App will be made in an HTML file. It will determine all things we want to show on our app. There will be an image of the song, the name of the song, the name of the artist and that is not it, there will be few buttons to play/pause and to go to previous/next songs. Finally linking our HTML file to the CSS file, the JavaScript file and not to forget, linking to the icon file inside the icon folder. To do that we need to simply link in the head of the HTML file by putting
<link rel="stylesheet" href="./icons/css/all.min.css" />
In the HTML file, everything is wrapped inside one another. There are various classes
and id
s which has the small chunks of the elements to be displayed. Put up some dummy data to form the layout of the app. For example:
<h2 id="song-title">Hello this is title</h2>
<h3 id="song-artist">This is artist/band name</h3>
Among the elements, there will be a separate div
tag which will hold up the image along with a class name which will come in handy while dealing with it in JavaScript.
<div id="song-image" class="song-img" style="background-image: url('./images/song-1.jpg');"></div>
There will many tags for each element requires like the name of the song and artist, to hold up the buttons, and also tags to wrap all the other elements. You can wish to think out of the box and add something more or change the arrangements.
NOTE: Now that we have linked the icons file, we can use the play and pause buttons, the previous and next buttons easily by simply adding their respective class names.
Code:
<body>
<div class="app-wrapper">
<div class="player">
<audio id="music-player"></audio>
<div id="song-image" class="song-img" style="background-image: url('./images/song-1.jpg');"></div>
<div class="song-details">
<h2 id="song-title">Hello this is title</h2>
<h3 id="song-artist">This is artist/band name</h3>
</div>
<div class="player-controls">
<button id="prev-btn" class="skip-btn"><i class="fas fa-backward fa-lg"></i></button>
<button id="play-btn" class="play"><i class="fas fa-play play-icon fa-2x"></i></i></button>
<button id="next-btn" class="skip-btn"><i class="fas fa-forward fa-lg"></i></button>
</div>
</div>
</div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css"></script>
<script src="./script.js"></script>
</body>
CSS Part:
Now that the HTML for the Music App is ready, add some CSS/styles to make it look good and place them in order so that it will look nice and clean. With proper CSS of own choice can give different results.
Example:
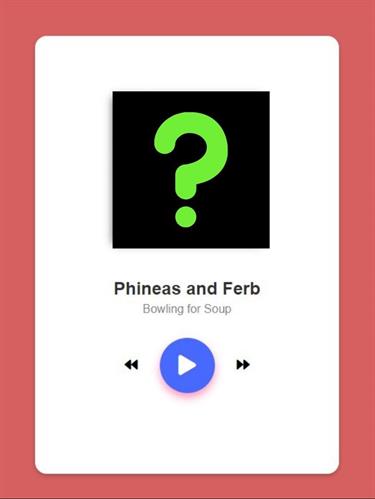
JavaScript Part:
First thing is that we want all the functioning of the app just right away when the window/page gets load so everything we will code in JavaScript will be inside window
an object triggered with the event forming an anonymous function.
window.onload=()=>{}
So inside this function, first select all the classes and ids from the HTML, to which we are going to make changes in a bit.
For example:
const song_img_el = document.querySelector('#song-image');
const song_title_el = document.querySelector('#song-title');
In this way, it will easier to point out which element we want to work with.
Add two variables, name them relevant to store the next and previous songs.
Creating a database for the app:
In the beginning, we created two folders named images and music, you put the images and songs in their respective folders. Make sure to name them numerically so that it will be easy to point them from the JavaScript.
To create the database, make an object of various properties of your songs and these properties will include:
- Name of the song
- Name of the artist/band
- Path of the song
- Path of the image of that song For example:
let songs = [
{
title: 'Wavin Flags',
artist: "K'naan",
song_path: 'music/Music-1.mpeg',
img_path: 'images/image-1.jpeg'
},
{
title: 'Phineas and Ferb ',
artist: "Bowling for Soup",
song_path: 'music/Music-2.mpeg',
img_path: 'images/image-2.jpeg'
},
}
Adding events and event-listeners
The next part is to make the app understand when to do things. Like if someone clicks on the play button then what to do?
All these are done with the help of calling functions when there is an event. Which can be triggered by event-listeners.
For example:
play_btn.addEventListener('click', TogglePlaySong);
In the above code when there will be an event with the play_btn
it will call the TogglePlaySong
function.
Code:
play_btn.addEventListener('click', TogglePlaySong);
next_btn.addEventListener('click', () => ChangeSong());
prev_btn.addEventListener('click', () => ChangeSong(false));
Defining the functions
Now that the database is ready and event-listeners are set, it is time to define the functions.
The functions will help to:
- When to play and pause
- Which song to play next
In this app I have used four functions to carry out the above functionalities.
<1> The first function is to play the song. Here the index of current song plus the next song will be collected and the UpdatePlayer
function will be called.
function InitPlayer () {
current_song_index = 0;
next_song_index = current_song_index + 1;
UpdatePlayer();
}
<2> The second function is to change the song when either the songs ends or the user clicks on the button of previous/next. This function will have a parameter of boolean value, which will see that if it is true the index of current song will be increased which means the next song or else the index will be decreased which means previous song. Along with this, it will also have a conditional rendering which will keep a track of the index number values, so that it will go on and on in a loop when the value exceeds or lesser than the possible index number of the database object.
At last it will also call the UpdatePlayer
functions.
if (current_song_index > songs.length - 1) {
current_song_index = 0;
next_song_index = current_song_index+1;
}
In the above code you can see that there is conditions which states that just in case where the index
of the list exceed the length of the number of songs then go back to index
0. Which means it will stay in a loop. Work on it and perform the basic idea to keep the music app in loops.
Code:
function ChangeSong (next = true) {
if (next) {
current_song_index++;
next_song_index = current_song_index + 1;
if (current_song_index > songs.length - 1) {
current_song_index = 0;
next_song_index = current_song_index+1;
}
if (next_song_index > songs.length - 1) {
next_song_index = 0;
}
} else {
current_song_index--;
next_song_index = current_song_index + 1;
if (current_song_index < 0) {
current_song_index = songs.length - 1;
next_song_index = 0;
}
}
UpdatePlayer();
TogglePlaySong();
}
<3> The third function will help to toggle between the play and pause button. This will make sure to change the button when the music plays or stops. This will be done by adding and removing class fro the HTML file with the help of JavaScript.
To add and remove class we can simple use element_name.classList.add('name_of_class')
and element_name.classList.remove('name_of_class')
respectively.
One more thing about this is that, we have to write the code in a vice-versa condition. Which means that if there is a play button then on click it will change to pause button and if it is a pause button, on click it will change to play button.
function TogglePlaySong () {
if (audio_player.paused) {
audio_player.play();
play_btn_icon.classList.remove('fa-play')
play_btn_icon.classList.add('fa-pause');
} else {
audio_player.pause();
play_btn_icon.classList.add('fa-play')
play_btn_icon.classList.remove('fa-pause');
}
}
<4> The fourth and last function which is the UpdatePlayer
function. This has a very crucial role in our app since its main job is to put up the current song name, image and details to our page and also to play the song too.
function UpdatePlayer() {
let song = songs[current_song_index];
song_img_el.style = "background-image: url('" + song.img_path + "');";
song_title_el.innerText = song.title;
song_artist_el.innerText = song.artist;
audio_player.src = song.song_path;
}