Consume ASP.NET Core Web API using HttpClient
HttpClient is a modern HTTP client for .NET applications. It can be used to consume functionality exposed over HTTP. For example, a functionality exposed by an ASP.NET Web API can be consumed in a desktop application using HttpClient. Using HttpClient you can send requests and receive responses using standard HTTP verbs such as GET, POST, PUT and DELETE. In this article, you will learn how to use HttpClient to consume ASP.NET Web API.
The following topics will be covered in this article.
- Convert Object to /from JSON
- Convert ListObjects to /from JSON
As of this writing, HttpClient is in the Beta stage and is available as a NuGet package. You must install the following NuGet packages before working with any of the examples discussed in this article:
- System.Net.Http: Provides the basic HttpClient and related classes
- System.Net.Http.Formatting: Support for serialization, deserialization, and additional features
- System.Json: Support for JsonValue, which is a mechanism for reading and manipulating JSON documents
Create ASP.NET Core Web API Project
On the Visual Studio, create a new ASP.NET Core Web Application project
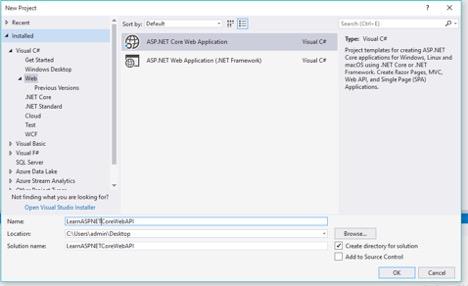
Select Empty Template
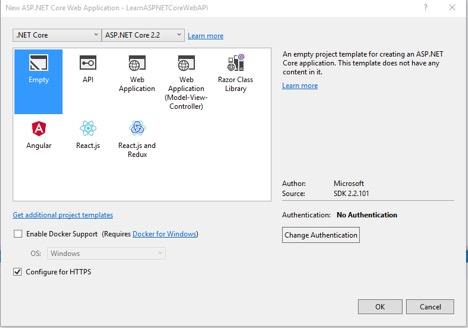
Click Ok button to Finish
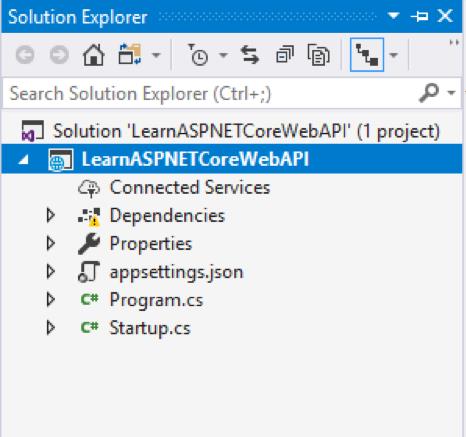
Add Configurations
Open Startup.cs file and add new configurations as below:
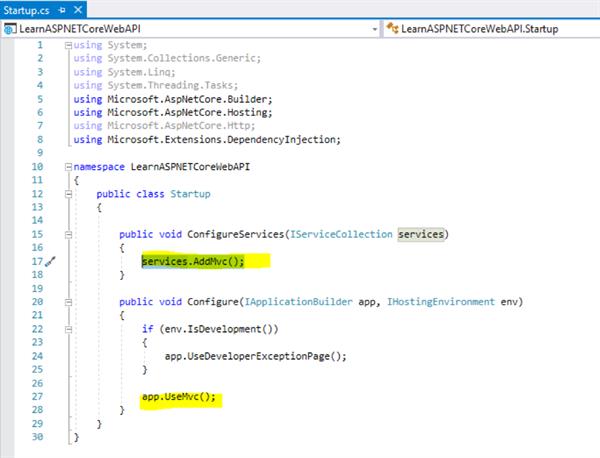
Here is the same code as showing in Image:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.Extensions.DependencyInjection;
namespace LearnASPNETCoreWebAPI
{
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseMvc();
}
}
}
Entities Class
Create a Models folder. In this folder, create a new class named Movie.cs as below:
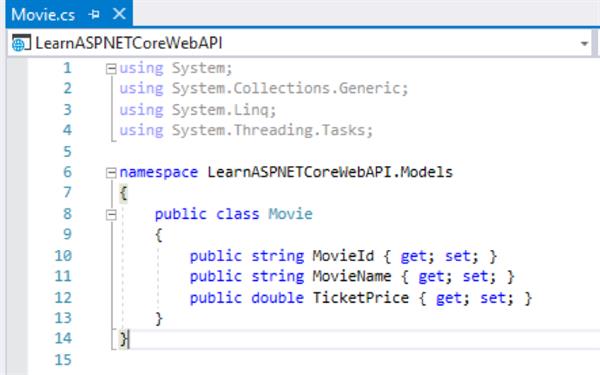
Movie Entity
Here is the same code as showing in Image:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace LearnASPNETCoreWebAPI.Models
{
public class Movie
{
public string MovieId { get; set; }
public string MovieName { get; set; }
public double TicketPrice { get; set; }
}
}
Create Controller
Create a new folder named Controllers. In this folder, create a new controller named MovieController.cs as below:
Action Method: Find Movie Details
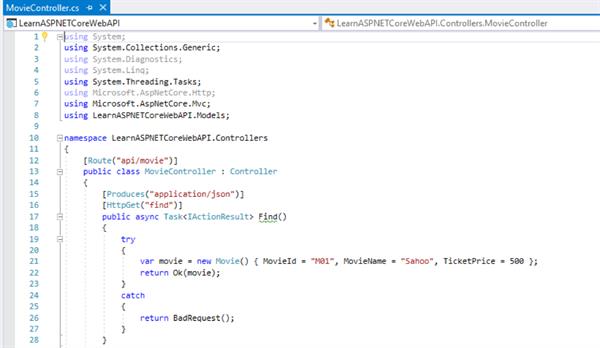
Action Method: Find all Movies Details
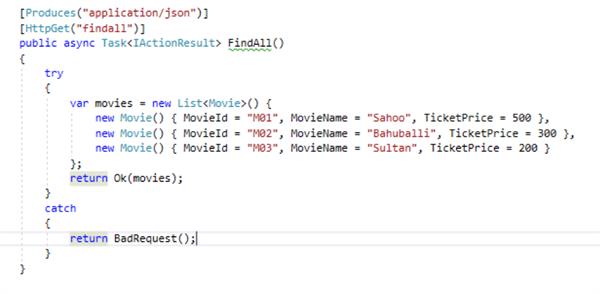
Here is the same code as showing in Image:
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using LearnASPNETCoreWebAPI.Models;
namespace LearnASPNETCoreWebAPI.Controllers
{
[Route("api/movie")]
public class MovieController : Controller
{
[Produces("application/json")]
[HttpGet("find")]
public async Task<IActionResult> Find()
{
try
{
var movie = new Movie() { MovieId = "M01", MovieName = "Sahoo", TicketPrice = 500 };
return Ok(movie);
}
catch
{
return BadRequest();
}
}
[Produces("application/json")]
[HttpGet("findall")]
public async Task<IActionResult> FindAll()
{
try
{
var movies = new List<Movie>() {
new Movie() { MovieId = "M01", MovieName = "Sahoo", TicketPrice = 500 },
new Movie() { MovieId = "M02", MovieName = "Bahuballi", TicketPrice = 300 },
new Movie() { MovieId = "M03", MovieName = "Sultan", TicketPrice = 200 }
};
return Ok(movies);
}
catch
{
return BadRequest();
}
}
}
}
Structure of ASP.NET Core Web API Project
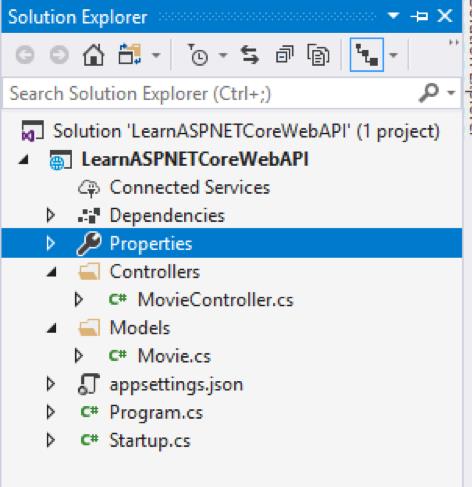
Now just Testing Web API to run in the local system.
Access Web API with using the following URL just change the port number:
https://localhost:44348/api/movie/find
Output
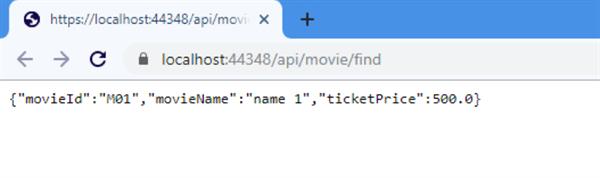
If you want to get all the data from API then call for findall Function using you URL, as follows:
/api/movie/findall

Now Consume your Web API into Console Application
Create a Console App (.NET Framework) Project in Visual Studio.
Entity Class
Create a Models folder in Console Application. In this folder, create a new class named Movie.cs as below:
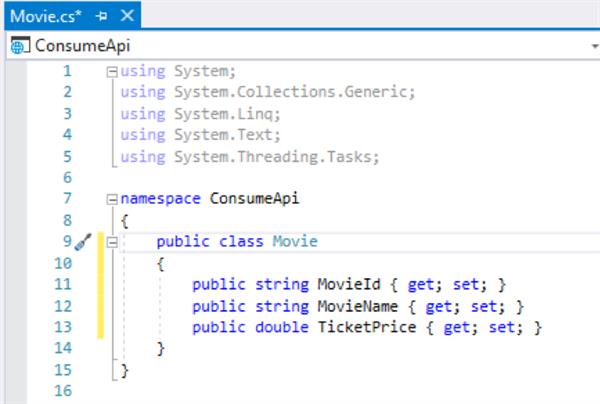
Movie Entity
Here is the same code as showing in Image:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsumeApi
{
public class Movie
{
public string MovieId { get; set; }
public string MovieName { get; set; }
public double TicketPrice { get; set; }
}
}
Create MovieRestClientModel
MovieRestClientModel class contains methods call Web API. Add a reference to System.Net.Http.Formatting library from Nuget Packages
Consuming MovieObject using HttpClient
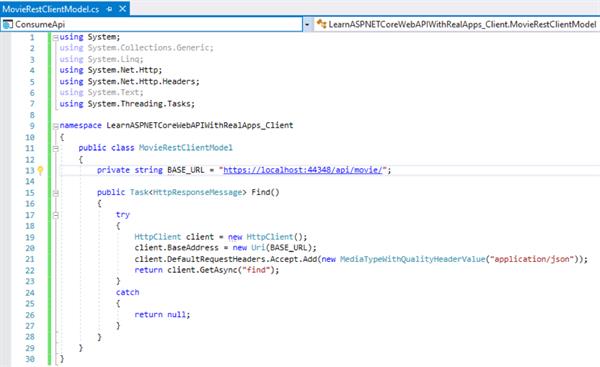
Consuming ListObject using HttpClient
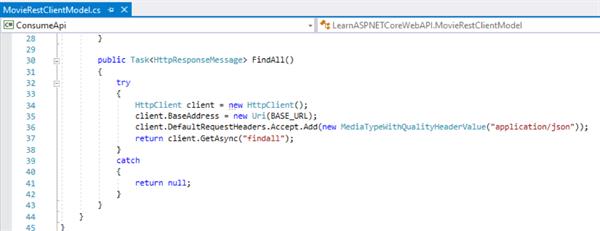
Here is the same code as showing in Image:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Threading.Tasks;
namespace LearnASPNETCoreWebAPIWithRealApps_Client
{
public class MovieRestClientModel
{
private string BASE_URL = "https://localhost:44348/api/movie/";
public Task<HttpResponseMessage> Find()
{
try
{
HttpClient client = new HttpClient();
client.BaseAddress = new Uri(BASE_URL);
client.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
return client.GetAsync("find");
}
catch
{
return null;
}
}
[Produces("application/json")]
[HttpGet("findall")]
public async Task<IActionResult> FindAll()
{
try
{
var movies = new List<Movie>() {
new Movie() { MovieId = "M01", MovieName = "Sahoo", TicketPrice = 500 },
new Movie() { MovieId = "M02", MovieName = "Bahuballi", TicketPrice = 300 },
new Movie() { MovieId = "M03", MovieName = "Sultan", TicketPrice = 200 }
};
return Ok(movies);
}
catch
{
return BadRequest();
}
}
}
}
Run your Console Application Now.
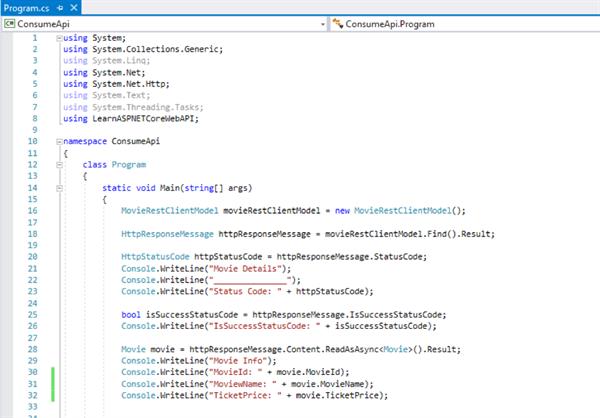
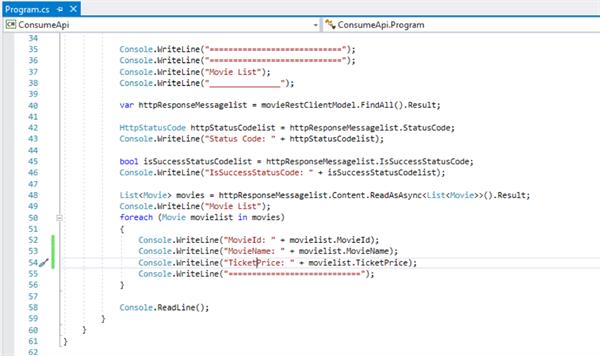
Here is the Full code as showing in Images:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using LearnASPNETCoreWebAPI;
namespace ConsumeApi
{
class Program
{
static void Main(string[] args)
{
MovieRestClientModel movieRestClientModel = new MovieRestClientModel();
HttpResponseMessage httpResponseMessage = movieRestClientModel.Find().Result;
HttpStatusCode httpStatusCode = httpResponseMessage.StatusCode;
Console.WriteLine("Movie Details");
Console.WriteLine("_______________");
Console.WriteLine("Status Code: " + httpStatusCode);
bool isSuccessStatusCode = httpResponseMessage.IsSuccessStatusCode;
Console.WriteLine("IsSuccessStatusCode: " + isSuccessStatusCode);
Movie movie = httpResponseMessage.Content.ReadAsAsync<Movie>().Result;
Console.WriteLine("Movie Info");
Console.WriteLine("MovieId: " + movie.MovieId);
Console.WriteLine("MoviewName: " + movie.MovieName);
Console.WriteLine("TicketPrice: " + movie.TicketPrice);
Console.WriteLine("============================");
Console.WriteLine("============================");
Console.WriteLine("Movie List");
Console.WriteLine("_______________");
var httpResponseMessagelist = movieRestClientModel.FindAll().Result;
HttpStatusCode httpStatusCodelist = httpResponseMessagelist.StatusCode;
Console.WriteLine("Status Code: " + httpStatusCodelist);
bool isSuccessStatusCodelist = httpResponseMessagelist.IsSuccessStatusCode;
Console.WriteLine("IsSuccessStatusCode: " + isSuccessStatusCodelist);
List<Movie> movies = httpResponseMessagelist.Content.ReadAsAsync<List<Movie>>().Result;
Console.WriteLine("Movie List");
foreach (Movie movielist in movies)
{
Console.WriteLine("MovieId: " + movielist.MovieId);
Console.WriteLine("MovieName: " + movielist.MovieName);
Console.WriteLine("TicketPrice: " + movielist.TicketPrice);
Console.WriteLine("============================");
}
Console.ReadLine();
}
}
}
your App Output must be like:
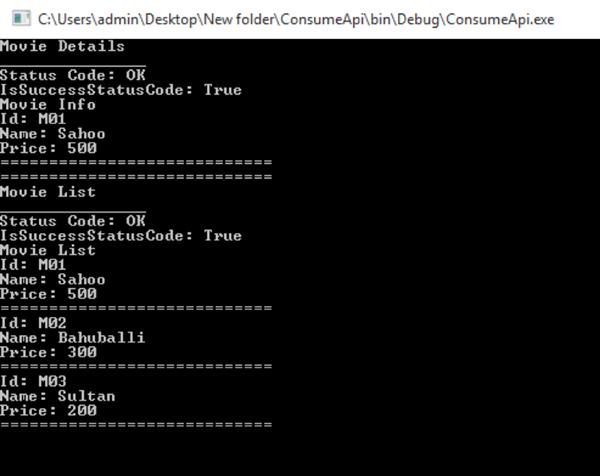