What do you mean by React Props Validation? | React Tutorial
What do you mean by React Props Validation? | React Tutorial
React Props Validation
Props are an important technique for passing the read-only attributes to React components. These are usually required to use correctly in the component. The components may not behave as expected If it is not used correctly. Hence, props are required to use props validation in improving react components.
Props validation is a tool. It will help the developers to avoid future bugs and problems. This is a useful way to force the correct usage of your components. This makes your code more readable. And these React components used special property PropTypes that help you to catch bugs by validating data types of values passed through props, although it is not necessary to define components with propTypes. However, it helps you to avoid unexpected bugs if you use propTypes with your components.
Validating Props
In the react component, App.propTypes is used for props validation. you will get the warnings on the JavaScript console Whenever some of the props are passed with an invalid type. it will be set to the App.defaultProps, After specifying the validation patterns.
Syntax:
class App extends React.Component {
render() {}
}
Component.propTypes = { /*Definition */};
ReactJS Props Validator
The below-given list is ReactJS props validator.
SN | PropsType | Description |
---|---|---|
1. | PropTypes.any | It can be of any data type. |
2. | PropTypes.array | This should be an array. |
3. | PropTypes.bool | This should be a boolean. |
4. | PropTypes.func | This should be a function. |
5. | PropTypes.number | This should be a number. |
6. | PropTypes.object | This should be an object. |
7. | PropTypes.string | This should be a string. |
8. | PropTypes.symbol | This should be a symbol. |
9. | PropTypes.instanceOf | It should be an instance of a particular JavaScript class. |
10. | PropTypes.isRequired | This must be provided. |
11. | PropTypes.element | This must be an element. |
12. | PropTypes.node | This can render anything like numbers, strings, elements, or an array (or fragment) containing these types. |
13. | PropTypes.oneOf() | This should be one of several types of specific values. |
14. | PropTypes.oneOfType([]) | This could be one of many types of an object. |
Example
In this example, we are creating an App component that contains all the props that we need. App.propTypes is used for props validation. For validating the props , we must have to add this line: import PropTypes from 'prop-types' in the App.js file.
App.js
import React, { Component } from 'react';
import PropTypes from 'prop-types';
class App extends React.Component {
render() {
return (
<div>
<h1>ReactJS Props validation example</h1>
<table>
<tr>
<th>Type</th>
<th>Value</th>
<th>Valid</th>
</tr>
<tr>
<td>Array</td>
<td>{this.props.propArray}</td>
<td>{this.props.propArray ? "true" : "False"}</td>
</tr>
<tr>
<td>Boolean</td>
<td>{this.props.propBool ? "true" : "False"}</td>
<td>{this.props.propBool ? "true" : "False"}</td>
</tr>
<tr>
<td>Function</td>
<td>{this.props.propFunc(5)}</td>
<td>{this.props.propFunc(5) ? "true" : "False"}</td>
</tr>
<tr>
<td>String</td>
<td>{this.props.propString}</td>
<td>{this.props.propString ? "true" : "False"}</td>
</tr>
<tr>
<td>Number</td>
<td>{this.props.propNumber}</td>
<td>{this.props.propNumber ? "true" : "False"}</td>
</tr>
</table>
</div>
);
}
}
App.propTypes = {
propArray: PropTypes.array.isRequired,
propBool: PropTypes.bool.isRequired,
propFunc: PropTypes.func,
propNumber: PropTypes.number,
propString: PropTypes.string,
}
App.defaultProps = {
propArray: [1,2,3,4,5],
propBool: true,
propFunc: function(x){return x+5},
propNumber: 1,
propString: "Tutorials link",
}
export default App;
Main.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App.js';
ReactDOM.render(<App/>, document.getElementById('app'));
Output:
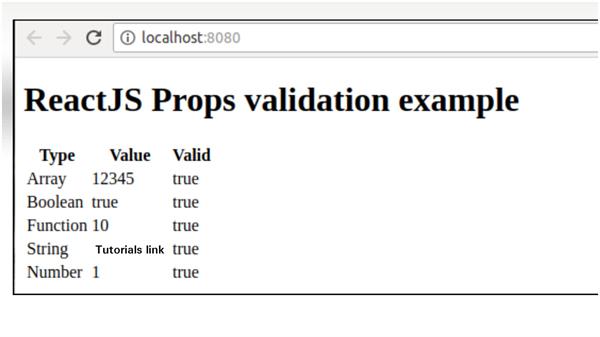
ReactJS permits creating a custom validation function to perform custom validation. The below-given argument is used to create a custom validation function.
props: This should be the first argument in the component.
propName: This is the propName that is going to validate.
componentName: This is the componentName that is going to validated again.
Example
var Component = React.createClass({
App.propTypes = {
customProp: function(props, propName, componentName) {
if (!item.isValid(props[propName])) {
return new Error('Validation failed!');
}
}
}
})